Datatables is a javascript plugin that allows for data to be presented in a flexible table with options for sorting and paginating the table. Once you get datatables working it opens up a range of functionality in terms of presenting tabular information. Although you can pass datatables a range of formats it works nicely with json.
To install Datatables using Node Package Manager type the following at the Command Line Interface
npm i datatables
To use laravel mix and webpack to bundle this up with your other assets, place the following line a the bottom of /resources/js/app.js
require('datatables');
Then for the css add the following line to the bottom of /resources/scss/app.scss
@import "~datatables/media/css/jquery.dataTables.css";
Now to build these two assets into /public/app.css and /public/app.js type the following command into the CLI
npm run dev
Now that Datatables have been bundled in, we can use that code from within Laravel Blade views. Before we can do that we need to get some json from that we can give the plugin to render. To get json from our tennisclub system, we need a new controller function and a matching route. Add the following function to /App/Http/Controllers/memberController.php
public function json()
{
//$this->view->disable();
$content = \App\Models\Member::all()->toJson();
return response($content)->withHeaders([
'Content-Type' => 'application/json',
'charset' => 'UTF-8'
]);
}
Add the following route which calls the controller function to /routes/web.php
Route::get('/members/all/datatables', 'App\Http\Controllers\memberController@datatables')->name('members.datatables');
Along with the controller function which returns json data we will need the controller function to return the view containing the datatables. Add the following function to \App\Http\Controllers\memberControllers
public function datatables()
{
return view('members.datatables');
}
And the following corresponding route to /routes/web.php
Route::get('/members/all/datatables', 'App\Http\Controllers\memberController@datatables')->name('members.datatables');
Finally to get it all working we need a view add the following code to new file called /resources/views/members/datatables.blade.php
@extends('layouts.app') @section('content') <table id="membertable" class="display" style="width:100%"> <thead> <tr> <th>MemberID</th> <th>Firstname</th> <th>Surname</th> <th>MemberType</th> </tr> </thead> </table> <script> $(document).ready(function () { $('#membertable').DataTable({ ajax: { url: '/members/all/json', dataSrc: "" }, columns: [ { data: 'id' }, { data: 'firstname' }, { data: 'surname' }, { data: 'membertype' } ], drawCallback: function () { rows = document.querySelectorAll('#membertable tbody tr'); [].forEach.call(rows, function(row) { }); } }); }); </script> @endsection
Now when you visit http://localhost:8000/members/all/datatables you see the members of the tennis club presented as follows
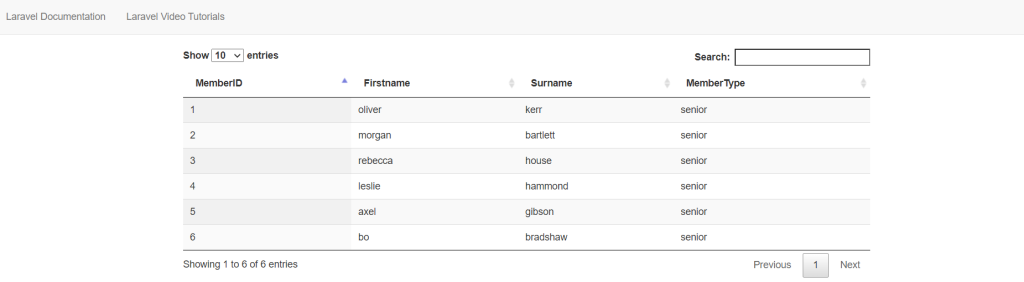