When the user uploads their various image files and submits the form the store() function of app/controllers/MemberimageController.php will be called. We now need to modify this function to allow it to process the array of files that will be uploaded. Modify the store() function that was generated by the scaffold replacing the code in the function with the code below.
if ($request->hasFile('imagefile')) { $files = $request->file('imagefile'); $i=0; foreach ($files as $file) { $memberimage = new \App\Models\Memberimages(); $memberimage->memberid = $request->memberid; $memberimage->imagefile = base64_encode(file_get_contents($file)); $memberimage->description = $request->description[$i]; if (!$memberimage->save()) { Flash::error('Error - File not saved to the DB'); } $i++; } }
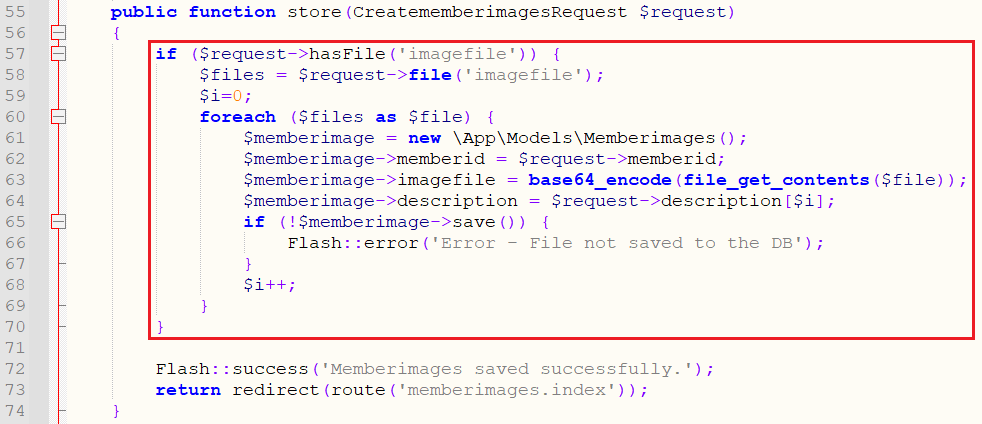
Now when you submit the form with the images they will be stored in base64 encoded form in the database.
The simplest modification we can make to display these images and verify that the uploads have worked correctly is to the memberimages index page. To make this update edit the file resources/views/memberimages/table.blade.php
Add an additional table header and a new table column to replace the existing memberimage column as follows.
<th>Image File</th>
and the column
<td> <img class="img-responsive center-block" height="200" width="100" src="data:image/jpeg;base64,{{$memberimages->imagefile}}"> </td>
Place these new snippets of code into the existing code as follows.
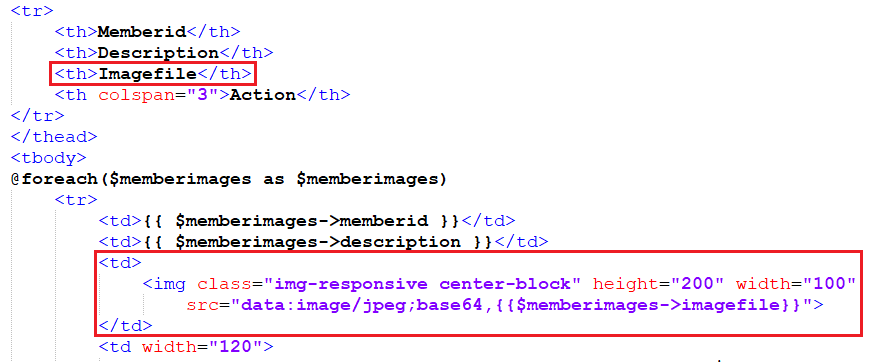
While this is useful to verify that the images are uploading correctly, what we really need is the ability to display the list of images associated with each member. To do this we're going to modify the show() and edit() functions of the Member so that the display all the images associated with that member in a carousel. We'll do this in the next post.