Laravel Breeze started development in 2011 as a package to provide a main set of functionality around user Login. More recently it has been folded in as the main approach to Laravel User Login and authentication as a "Starter Kit". The default set of views around this are based on blade styled with tailwind.css. In addition developers can choose to use ReactJs or Vue templates. We're going to keep it as simple as possible to start with.
Before we can use any of this great functionality, we need a set of database tables in which to store information related to user login and authentication. A set of these tables has been provided for us in the form of database migrations. A database migration is a PHP script which will create(or modify) a database table for us. These files were created and stored in database\migration at the time your project was created. To use them type the following into your project root folder prompt in the CLI
php artisan migrate

This will generate a number of tables - everything we need to support the basic functionality around login and authentication
users
password_resets
personal_access_tokens
In addition, there are two tables created to help keep track of migrations - migrations, and failed_jobs. Once that's complete we can go ahead and install Breeze. To download the package type
php composer.phar require laravel/breeze:1.9.2
Before we actually install breeze we have to do a bit of housekeeping. The way breeze installs it creates a brand new routes/web.php file. This is not ideal as it will wipe out any existing routes you have created/generated. To deal with this, back up your existing routes/web.php file - after Breeze has been installed you can copy your routes from your backup file back into the routes/web.php file at the bottom of this new web.php. Take care to place your old routes at the BOTTOM of the file. The Laravel Breeze installer has added a crucial line require DIR.'/auth.php'; which pulls in a load of new routes associated with authorisation and login. We don't want to overwrite this line and not get these new routes.
In addition to nuking the routes/web.php the Breeze installer also creates a new resources/layouts/app.blade.php file. Given this, you should also back up (take a copy of) the resources/layouts/app.blade.php file. After the Breeze installer has completed, you can replace the entire app.blade.php file which has newly been generated with your original one. Once you've backed up these two files you can go ahead and install Breeze. To do this type
php artisan breeze:install
Having run the migration and created the users table to support our login functionality, we need to manage one potential slight discrepency. Since Laravel v 5.4, Laravel uses the utf8mb4 character set to store data. This allows for longer string data and has support for, among other things, emojis to be stored in the database. The only issue is that older databases or versions of MySQL such as MariaDB may not support the longer String lengths. To account for this potential problem, open the file app/providers/AppServiceProvider.php and add the following two lines highlighted in red.

This will run whatever migration scripts that are stored in the database\migrations folder as follows
Note that there were, in fact, a number of tables created. Managing users involves keeping track of a range information including a history of password resets. In addition to creating tables the migration process also keeps track of what migration scripts and changes have been run. To do this it will generate a database table called migrations to store this information and a failed_jobs table to keep a log of anything that didn't work. Go to your preferred database client (in my case HeidiSQL) and check to enusre these new tables have been created.
User Login functionality as a whole incoroporates a whole range of different functions, from registering as a new user, to logging in and logging out but also password resets and reminders. Laravel Breeze added a range of routes associated with these functions in routes\auth.php. The last of these routes is the logout route. This route is available only as a Post Request. This approach is technically correct. Logout should really be implemented as a logout form - it's like asking the user "are you sure". After all there may be a lot of data stored in the session that the User has entered that will get lost when the user logs out. While setting up and testing the login function it is hand to have a logout route which can be called directly via a url Get Request. To achieve this we'll make a distinction from the logout route which is already there and call our route 'logoff'. To add this route add the following line to the bottom of routes\auth.php
Route::get('logoff', [AuthenticatedSessionController::class, 'destroy'])->name('logoff');
To see the effect of this single additional line of code go to the CLI and type in the command to show all available routes
php artisan route:list
You should see the following new routes related to authentication
Now to try out the new authentication functionality visit http://localhost:8000/register to register a new user in your application. Don't forget to ensure your webserver is running by typing php artisan serve at the CLI.
Once you have registered your user, go back and login using the username and password you have just created. Do this by visiting http://localhost:8000/login
The Laravel Breeze login redirects you to a the homepage once you are logged in. The home variable is set in app\providers\RouteServiceProvider.php. By default laravel breeze sets the home route as dashboard. You can reset/modify this by changing the home const variable and setting it to something more meaningful. For the moment maybe set it to /members as follows.
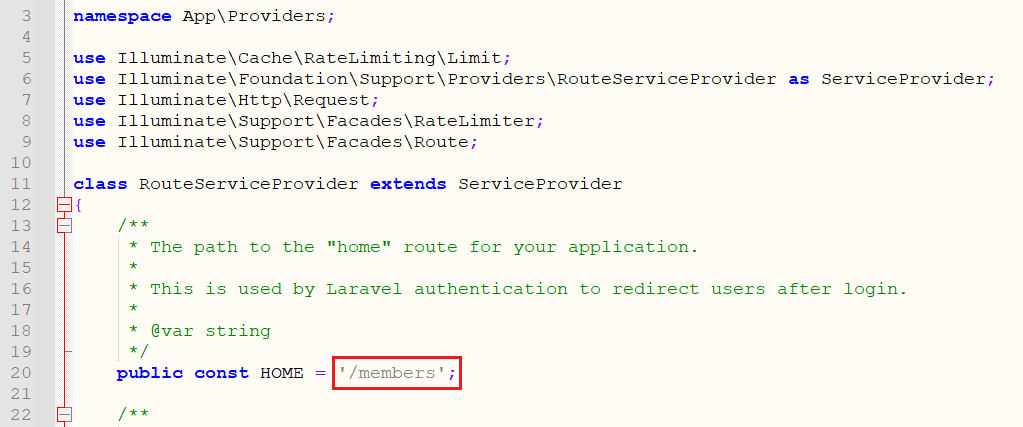